Using CircleCI to test and deploy Python serverless functions on Microsoft Azure
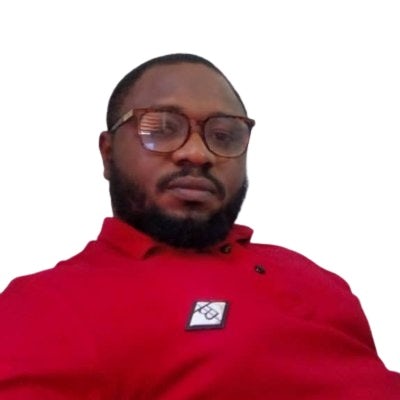
Fullstack Developer and Tech Author
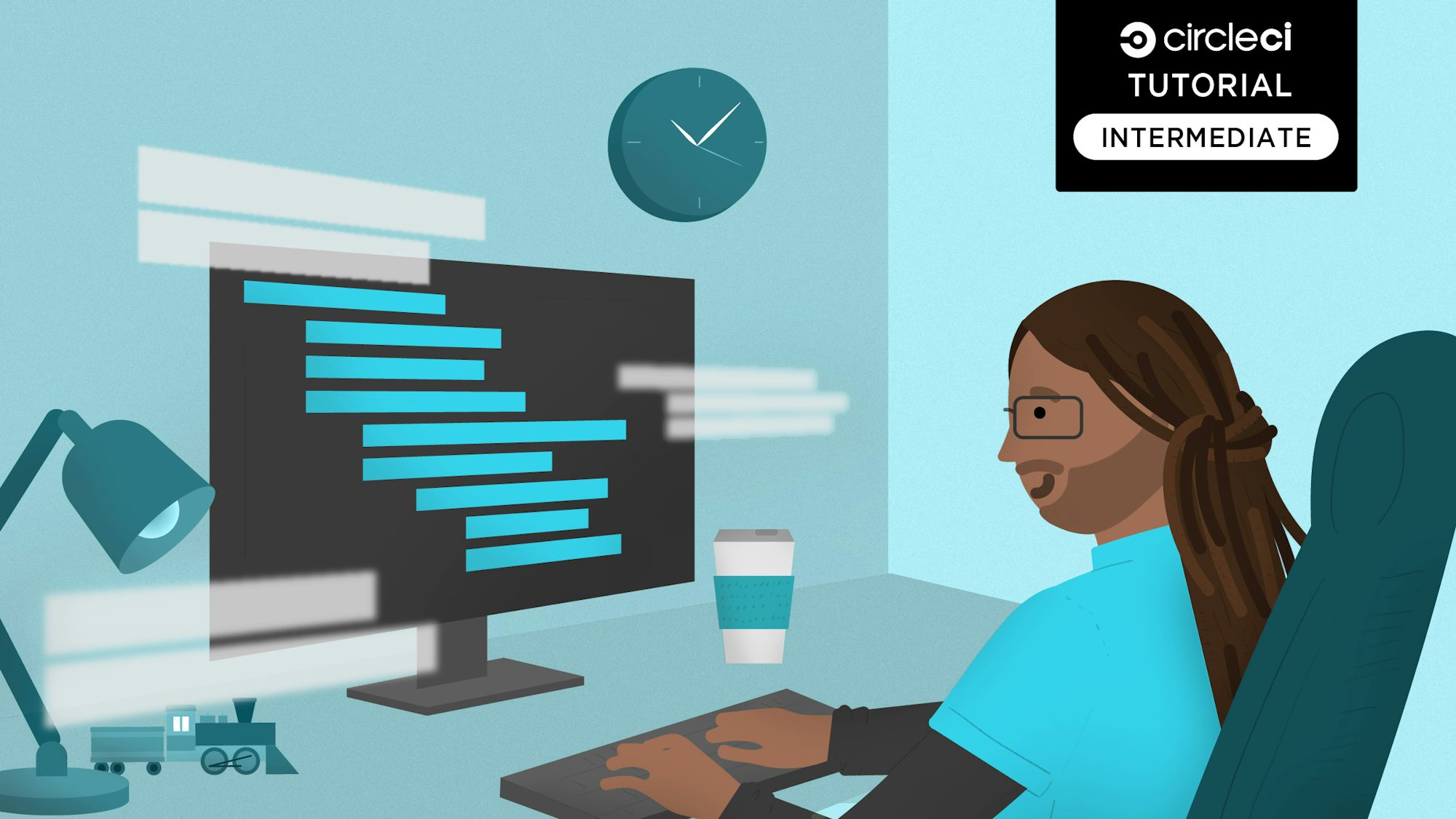
Serverless computing simplifies app development by abstracting away server management. Azure Functions provides a robust platform for event-driven, on-demand code execution. In this tutorial, we’ll create and deploy a Python-based Azure Function—one that parses incoming JSON—using CircleCI. For a more granular and enable programmatic access to Azure resources, we’ll use service principal for secure authentication and the Azure CLI orb to streamline our CI/CD pipeline.
By the end, you’ll have a reliable workflow that tests your function code, builds it, and deploys it to Azure — automatically.
Prerequisites
The following tools and accounts are required to follow this tutorial:
- Microsoft Azure Account with an active subscription
- Azure CLI installed locally
- Azure Functions Core Tools Select Python as the programming language (optional for local function testing)
- Python 3.9+
- GitHub account
- CircleCI Account
Setting up Azure for serverless functions
Before creating the Azure function, we need to set up the Azure environment. This includes creating a Resource Group, Storage Account, and a service principal for secure deployment.
To begin, ensure that you are authenticated with Azure CLI:
az login
Then issue the following command to created a Resource Group to logically group your Azure resources:
az group create --name MyResourceGroup --location eastus
Ensure to replace MyResourceGroup
and eastus
with names and locations that suit you.
Next, create a storage account to store metadata and logs:
az storage account create \
--name mypythonfuncstorage \
--resource-group MyResourceGroup \
--location eastus \
--sku Standard_LRS
This command creates a storage account named mypythonfuncstorage
in the MyResourceGroup
resource group. This is important for Azure Functions to store metadata and logs.
The --sku Standard_LRS
flag specifies the storage account type as Standard Locally Redundant Storage
. Find more details about Azure Storage Account types.
Creating Azure service principal
Microsoft Azure recommends using a service principal account for accessing Azure resources when automating or non-interactive scenarios. This is because service principals are identities created for use with applications, hosted services, and automated tools. They are used to access resources securely.
To create a service principal, run the following command:
az ad sp create-for-rbac --name "circleci-deployer" --role contributor \
--scopes /subscriptions/<your-subscription-id>
Ensure to replace <your-subscription-id>
with your Azure subscription ID.
Azure returns credentials like:
{
"appId": "xxxxx",
"password": "xxxxx",
"tenant": "xxxxx"
}
Later on, we’ll use these in CircleCI as AZURE_CLIENT_ID
, AZURE_CLIENT_SECRET
, AZURE_TENANT_ID
respectively to securely deploy our function.
Creating and testing the serverless function locally
Here, to keep things simple, we’ll write a simple Python HTTP triggered function that returns a personalized response. We’ll test it locally before deploying to Azure.
Use the installed Azure functions core tools to scaffold a new Python Azure Function in an empty directory:
func init --worker-runtime python
func new --name helloFunction --template "HTTP trigger"
When prompted to select Select a number for Auth Level
, choose 2
for Anonymous
, as authentication is not required for this function.
Select a number for Auth Level:
1. FUNCTION
2. ANONYMOUS
3. ADMIN
Choose option: 2
Appending to /Users/yemiwebby/tutorial/circleci/python-azure-hello-func/function_app.py
The function "helloFunction" was created successfully from the "HTTP trigger" template.
You’ll get a folder structure like the one below:
|-- host.json
|-- local.settings.json
|-- requirements.txt
|-- function_app.py
Here’s a brief description of each file:
host.json
– Configures global settings for the Azure Functions runtime.local.settings.json
– Stores local environment variables.requirements.txt
– This file lists Python dependencies for the function app.function_app.py
– Contains the function app code and function definitions.
The function code
Open the function_app.py
file and the content in the file should look like this:
import azure.functions as func
import datetime
import json
import logging
app = func.FunctionApp()
@app.route(route="helloFunction", auth_level=func.AuthLevel.ANONYMOUS)
def helloFunction(req: func.HttpRequest) -> func.HttpResponse:
logging.info('Python HTTP trigger function processed a request.')
name = req.params.get('name')
if not name:
try:
req_body = req.get_json()
except ValueError:
pass
else:
name = req_body.get('name')
if name:
return func.HttpResponse(f"Hello, {name}. This HTTP triggered function executed successfully.")
else:
return func.HttpResponse(
"This HTTP triggered function executed successfully. Pass a name in the query string or in the request body for a personalized response.",
status_code=200
)
This file defines an HTTP-triggered Azure Function using the azure.functions
module. It initializes a FunctionApp
instance and registers helloFunction
as a route at /api/helloFunction
, allowing anonymous access. The function extracts a name
parameter from the query string or request body, logs the request, and responds with a personalized greeting if a name is provided. If no name is found, it returns a default message prompting the user to include one.
Running the function locally
To test the function locally, run:
func start
You should see a similar output:
Found Python version 3.10.16 (python3.10).
Azure Functions Core Tools
Core Tools Version: 4.0.6821 Commit hash: N/A +c09a2033faa7ecf51b3773308283af0ca9a99f83 (64-bit)
Function Runtime Version: 4.1036.1.23224
[2025-03-17T11:45:57.716Z] Worker process started and initialized.
Functions:
helloFunction: http://localhost:7071/api/helloFunction
For detailed output, run func with --verbose flag.
[2025-03-17T11:46:02.696Z] Host lock lease acquired by instance ID '000000000000000000000000F151C0A9'.
Writing unit tests
Let’s write a unit test for the function. Create a test_helloFunction.py
file and add the following code:
import json
import unittest
from unittest.mock import MagicMock, patch
import azure.functions as func
from function_app import helloFunction
class TestHelloFunction(unittest.TestCase):
@patch('function_app.logging.info') # Mock logging to suppress log messages
def test_helloFunction_query(self, mock_log):
"""Test function when name is provided as a query parameter."""
req = func.HttpRequest(
method='GET',
url='/api/helloFunction',
params={'name': 'TestUser'},
body=None
)
resp = helloFunction(req)
self.assertEqual(resp.status_code, 200)
self.assertIn("Hello, TestUser.", resp.get_body().decode())
@patch('function_app.logging.info')
def test_helloFunction_body(self, mock_log):
"""Test function when name is provided in the JSON request body."""
req_body = json.dumps({"name": "JsonUser"}).encode('utf-8')
req = func.HttpRequest(
method='POST',
url='/api/helloFunction',
body=req_body,
headers={"Content-Type": "application/json"}
)
resp = helloFunction(req)
self.assertEqual(resp.status_code, 200)
self.assertIn("Hello, JsonUser.", resp.get_body().decode())
@patch('function_app.logging.info')
def test_helloFunction_invalid_json(self, mock_log):
"""Test function when the request body contains invalid JSON."""
req = func.HttpRequest(
method='POST',
url='/api/helloFunction',
body=b'{"name": "Incomplete',
headers={"Content-Type": "application/json"}
)
resp = helloFunction(req)
self.assertEqual(resp.status_code, 200)
self.assertIn("This HTTP triggered function executed successfully.", resp.get_body().decode())
if __name__ == '__main__':
unittest.main()
The unit test verifies the behavior of the helloFunction
endpoint using the unittest
framework. It mocks the logging calls to avoid cluttering test output. The first test checks if the function correctly processes a query parameter by simulating a GET request with a name
in the URL. The second test validates that the function extracts the name from a JSON request body in a POST request. Lastly, the third test ensures that if the request body contains invalid JSON, the function still responds gracefully with a default success message.
To run the test locally, create a virtual environment and install the required dependencies using the following command:
python -m venv .venv
source .venv/bin/activate
pip install -r requirements.txt
and then run the test with:
python -m unittest
If all tests pass, your function is ready for deployment.
...
----------------------------------------------------------------------
Ran 3 tests in 0.001s
OK
Create a function app in Azure
Now that we have our function ready, we can deploy it to Azure. We’ll create a Function App in the Consumption Plan (pay-per-execution) with the following command:
az functionapp create \
--resource-group MyResourceGroup \
--consumption-plan-location eastus \
--os-type Linux \
--runtime python \
--functions-version 4 \
--name circleciPythonFunctionApp \
--storage-account mypythonfuncstorage
This command creates a Function App named circleciPythonFunctionApp
in the MyResourceGroup
resource group. The --runtime python
flag specifies that the function app will run Python functions.
Ensure to replace MyResourceGroup
, mypythonfuncstorage
, and circleciPythonFunctionApp
with names that suit you.
In the next section, we’ll set up continuous integration with CircleCI to automate testing and deployment.
Setting up continuous integration and deployment with CircleCI
Create a .circleci/config.yml
file in the root of your project and add the following configuration script to it:
version: 2.1
orbs:
azure-cli: circleci/azure-cli@1.3.2
node: circleci/node@7.1.0
jobs:
test:
docker:
- image: cimg/python:3.9
steps:
- checkout
- run:
name: Install Dependencies
command: pip install -r requirements.txt
- run:
name: Run Tests
command: python -m unittest
deploy:
executor: node/default
steps:
- checkout
- azure-cli/install
- azure-cli/login-with-service-principal:
azure-sp: AZURE_CLIENT_ID
azure-sp-password: AZURE_CLIENT_SECRET
azure-sp-tenant: AZURE_TENANT_ID
- run:
name: Install Azure Functions Tools
command: npm install -g azure-functions-core-tools@4 --unsafe-perm
- run:
name: Publish to Azure Function App
command: func azure functionapp publish circleciPythonFunctionApp --python
workflows:
version: 2
test-and-deploy:
jobs:
- test
- deploy:
requires:
- test
In the configuration above, CircleCI automates testing and deployment of the Azure Function App. The test job runs in a Python 3.9 Docker container, installs dependencies, and executes unit tests. If tests pass, the deploy job checks out the code, installs Azure CLI
, logs into Azure using a service principal, and installs Azure Functions Core Tools. It then deploys the function using func azure functionapp publish
. The workflow ensures deployment only happens if tests pass.
Save all the changes and push the project to GitHub.
Now, go to the Projects page on the CircleCI dashboard. Select the associated GitHub account to add the project and click Set Up Project. You will be prompted to enter the branch housing your configuration file. CircleCI will detect the .circleci/config.yml
file and start building the project.
This will trigger the pipeline, but it will fail because we haven’t set up the environment variables for the required credentials in CircleCI yet:
Setting up environment variables in CircleCI
Click on the project settings in CircleCI and navigate to the Environment Variables section. Add the following environment variables:
- AZURE_CLIENT_ID (Service Principal App ID)
- AZURE_CLIENT_SECRET (Service Principal Password)
- AZURE_TENANT_ID (Service Principal Tenant ID)
Now, re-run the pipeline, and it should pass successfully.
The function is now deployed to Azure. Visit the deployed function URL to test it. In our case, the URL is https://circlecipythonfunctionapp.azurewebsites.net/api/hellofunction
Conclusion
This setup ensures your Python-based Azure Function is tested and deployed automatically using CircleCI. Secure credentials, and unit tests help maintain reliability. Each commit triggers the pipeline, enabling continuous delivery of the function to Azure. Enjoy a streamlined serverless workflow with CircleCI and Azure Functions!
The complete code for this tutorial is available on GitHub.