Implementing DevOps practices in front-end development
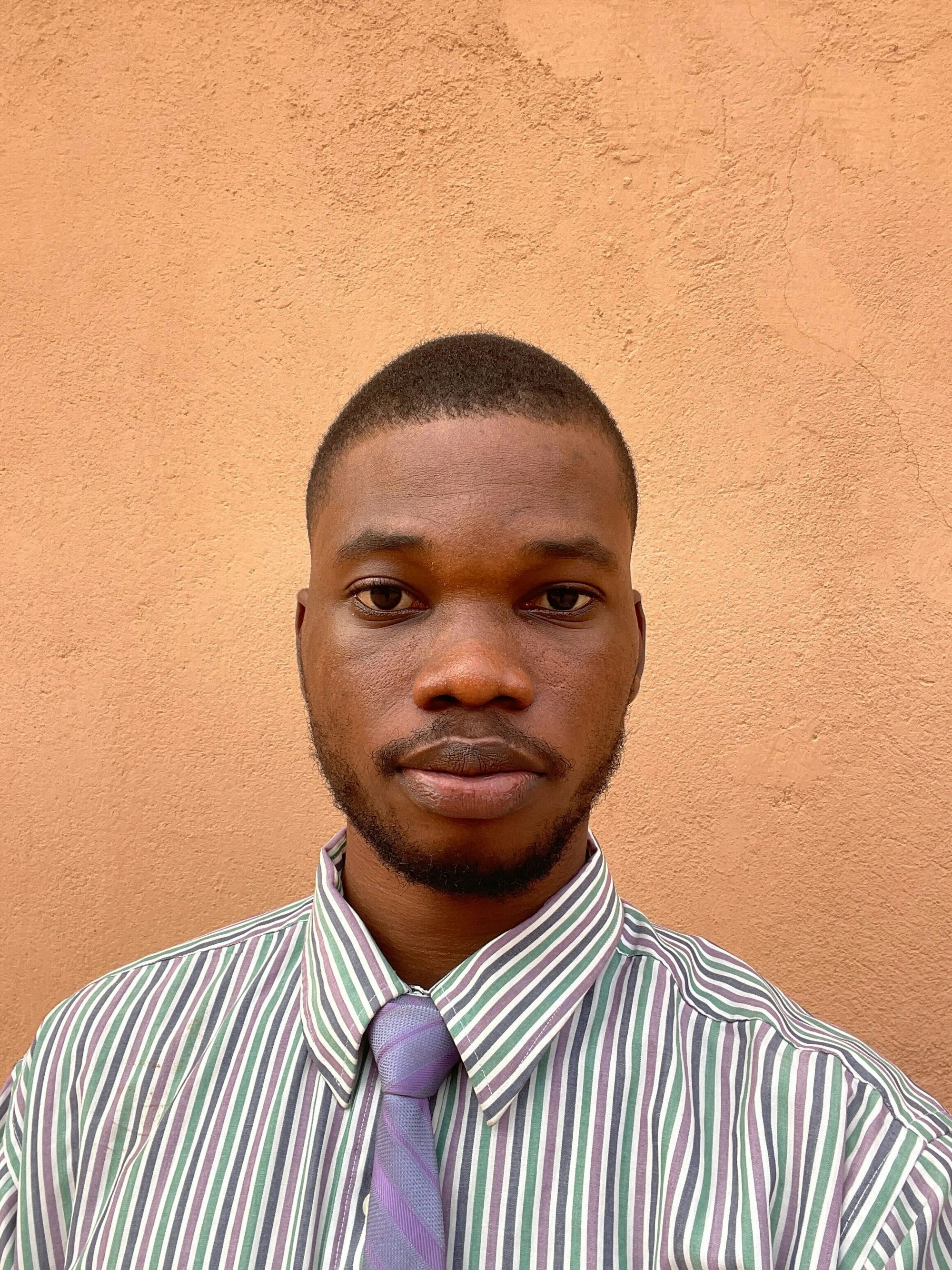
Front-end Developer
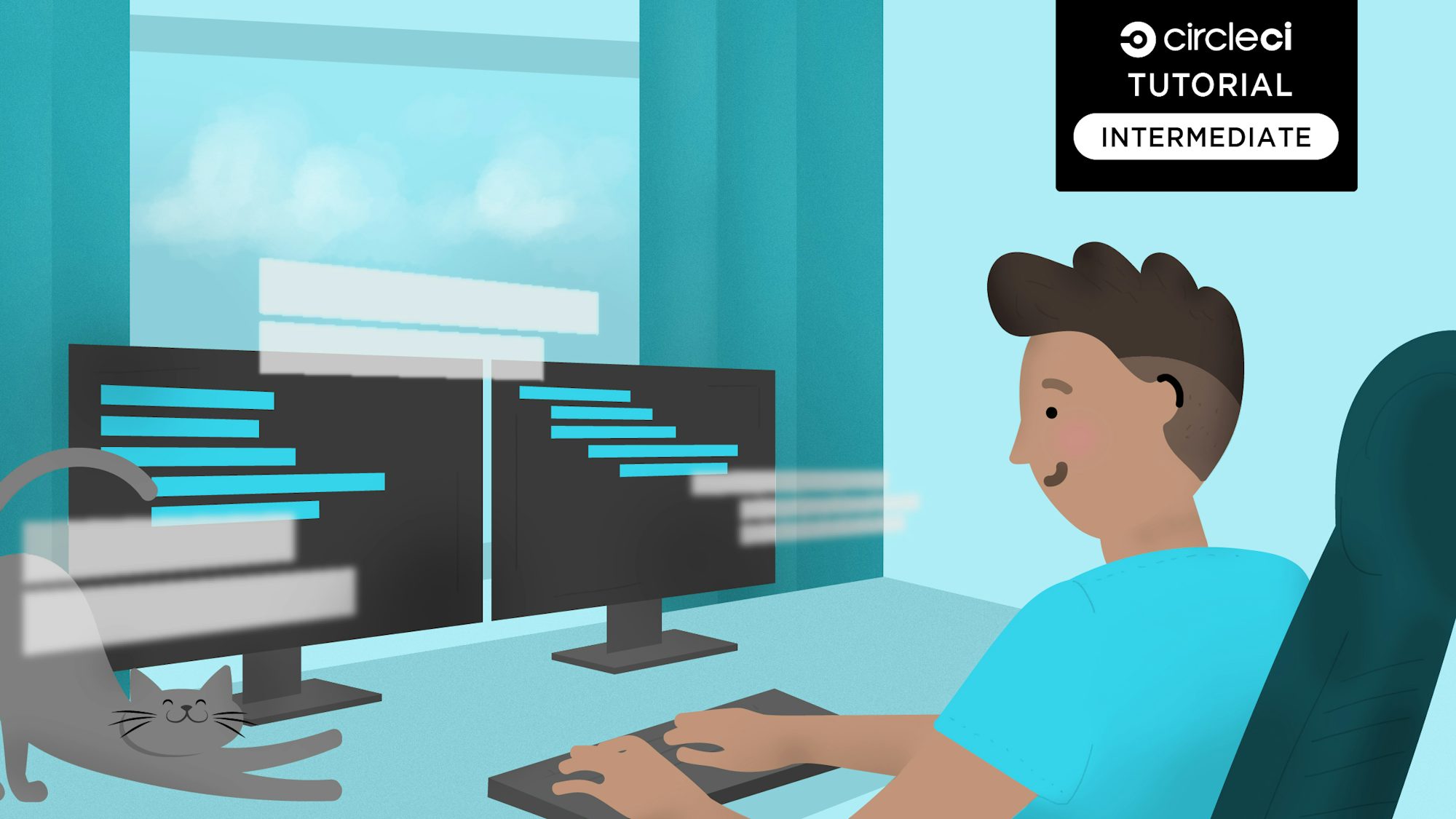
CI/CD is an approach to software development that combines continuous integration (CI) and continuous delivery (CD). CI/CD makes developing apps faster, safer, and more efficient. In front-end development, CI/CD becomes even more valuable as developers frequently test, build, and deploy applications. Automating this process reduces the need for developers to manually carry out these tasks, boosting efficiency and reliability.
CI is a modern DevOps practice that allows developers to automatically integrate and test code changes in software projects. On the other hand, CD allows tested code to be automatically released to production environments. By implementing both practices, front-end developers can achieve automated testing, faster and more reliable deployments, code consistency, visual regression testing, and performance monitoring.
In this article, you will learn how to set up a CI/CD pipeline using Lighthouse CI for automated performance and accessibility testing, Percy for automatically catching visual regression, and CircleCI as the automation tool to handle everything from integration to deployment. You will also learn how to configure CircleCI to handle automatic deployments to Vercel. This tutorial uses a simple React application to demonstrate the process, but you can use any front-end project you like.
Prerequisites
To follow this tutorial, you require:
- A basic knowledge of DevOps and front-end development.
- A GitHub account.
- A CircleCI account.
- Lighthouse CI.
- Node.js.
- A Percy account.
- A Vercel account.
Getting started with frontend project setup
To set up your CI/CD pipeline, you will need a front-end project. If you already have one that you would like to implement a CI/CD pipeline on, then that’s perfect. However, if you do not, here are the steps to create a simple React application.
Note: To create a React application, you need to have Node.js installed on your computer. To install Node, visit the official Node.js website. It is recommended to use Node.js version 14 or later for better compatibility.
Start by creating a project directory. This folder is where your React application would reside. For this tutorial, name your folder my-react-project
. Create and navigate into it using the following command:
mkdir my-react-project
cd my-react-project
Next, create your React application using Vite. To do this, run the below command into your terminal:
npm create vite@latest .
Running the above command in your terminal will prompt you to:
- Select a framework: choose React
- Select a variant: choose JavaScript
Next, install the necessary dependencies and files by entering the below command in your terminal:
npm install
Now that you have successfully created the React app, it’s time to run it in your browser. Enter this command into your terminal:
npm run dev
The above command will open a local URL in your terminal. Hold CTRL and click on the URL http://localhost:5173/
to launch your React app in your default browser. You will see a standard React welcome screen, which confirms that everything is set up correctly up.
You just succeeded in creating a simple React application. This will serve as the front-end project you will use to implement the CI/CD pipeline in the next steps.
Integrating CircleCI into your frontend project
To implement the CI/CD pipeline in this front-end project, you will be using CircleCI as your automation tool. This implies CircleCI will handle automated testing, building, and deployment of your front-end project whenever changes are made to the code base.
To begin, create a .circleci
directory at the root of your project. Next, create a config.yml
inside the directory and use the following content for it:
version: 2.1
orbs:
browser-tools: circleci/browser-tools@1.2.3
jobs:
build:
docker:
- image: cimg/node:20.9-browsers
working_directory: ~/your-project
steps:
- checkout
- browser-tools/install-chrome
- run:
name: Install Dependencies
command: npm install
- run:
name: Start Vite Dev Server
command: npm run dev
background: true
If you are new to CircleCI and creating a project for the first time, follow the steps in this guide to Create a Project in CircleCI and authorize CircleCI to access your GitHub, Bitbucket, or GitLab account. This will enable CircleCI to automatically detect your front-end project and set up a CI/CD pipeline for it. This tutorial uses GitHub as the version control system.
Next push the front-end project you want to set a CI/CD pipeline on to GitHub. This is necessary because CircleCI directly integrates with GitHub, meaning your project needs to be version-controlled with Git.
From your CircleCI dashboard, search for the my-react-app
project and click Set Up Project.
From the next screen, select the Fastest
option and enter the branch where your configuration file is located. In this tutorial, the branch is main
.
Click Set Up Project to complete the setup. And this should trigger the pipeline and build successfully.
Viola! You just succeeded in adding a CI/CD pipeline to your front-end project using CircleCI.
Lighthouse CI: Automating performance and accessibility testing
With CircleCI now set up to automate the building, testing, and deployment of Your front-end project, the next step is to enhance your workflow by integrating Lighthouse CI for automatic performance and accessibility testing. This implementation ensures that your frontend project not only functions correctly but also meets key performance and accessibility standards before deployment.
Setting up lighthouse CI is quite straightforward. To integrate Lighthouse CI, start by installing Lighthouse CI in your project and as a development dependency:
npm install --save-dev @lhci/cli@0.14.x
Next, create a configuration file named lighthouserc.cjs
at the root of your repository. You can either do this manually or by entering the below command in your terminal:
touch lighthouserc.cjs
Next, copy the following code into your created lighthouserc.cjs
configuration file:
module.exports = {
ci: {
collect: { url: ["http://localhost:5173"] },
assert: {
assertions: {
"categories:seo": "off",
"categories:best-practices": "off",
"categories:pwa": "off",
"categories:performance": ["error", { minScore: 0.6 }],
"categories:accessibility": ["error", { minScore: 0.8 }],
},
},
upload: {
target: "temporary-public-storage",
},
},
};
This file configures Lighthouse CI to run performance and accessibility tests on your front-end project. The collect: {url[]}
, specifies the URL of the project you want to test, the assertions: {}}
command specifies the type of test you want Lighthouse CI to run on your project while the upload:{target: 'temporary-public-storage'}
settings, uploads the Lighthouse CI report to a temporary publicly accessible storage, making it easier to share and review results.
Note: since this article focuses on utilizing Lighthouse CI for performance and accessibility testing, all other assertion categories like SEO, best practices, and PWA are set to off.
With your lighthouse configuration all set up, it’s time to modify your CircleCI configuration file to run Lighthouse CI. Update your CircleCI config.yml
to match the following configuration:
version: 2.1
orbs:
browser-tools: circleci/browser-tools@1.2.3
jobs:
build:
docker:
- image: cimg/node:20.9-browsers
working_directory: ~/your-project
steps:
- checkout
- browser-tools/install-chrome
- run:
name: Install Dependencies
command: npm install
- run:
name: Start Vite Dev Server
command: npm run dev
background: true
- run:
name: Run Lighthouse CI
command: npx lhci autorun
The above file first installs Chrome using the browser-tools/install chrome
command. The npx lhci autorun
command runs Lighthouse CI automatically.
Pushing these changes to GitHub triggers CircleCI, which then executes Lighthouse CI as part of its pipeline.
With Lighthouse CI successfully running on your CircleCI pipeline, Here is the performance and accessibility test of your front-end project.
Percy: Catching visual regressions automatically
Visual regression testing is a method that checks whether visual changes in an application introduce unintended differences. A common tool for this test is Percy. When visual differences are detected, Percy highlights them for review. In situations where changes are unintended, and could negatively impact a site, developers can prevent deployment until the issue is resolved.
Going forward, you will integrate Percy into your CircleCI pipeline to catch visual regressions automatically.
Note: Because this front-end project is a simple React application, you will integrate Percy with Cypress. Cypress is a popular end-to-end testing framework for React applications.
Setting up Percy
Start by installing Percy for Cypress:
npm install --save-dev @percy/cli @percy/cypress
Next, initialize Cypress by entering the following command into your terminal:
npx cypress open
This command will open the Cypress Test Runner. Select E2E Testing and follow the prompt to create a new test spec. This will generate the necessary Cypress configuration files in your front-end project.
To enable Percy in Cypress, add the following statement to your cypress/support/e2e.js
file:
import "@percy/cypress";
Next, copy the below code into your cypress/e2e/spec.cy.js
file:
describe("Percy Visual Test", () => {
it("should take a Percy snapshot", () => {
cy.visit("http://localhost:5173");
cy.percySnapshot("Homepage");
});
});
Note: Ensure you replace http://localhost:5173
with your project’s actual URL.
Now, run Percy locally to verify that it works:
npx percy exec -- cypress run
This command captures visual snapshots in your local development environment. Running Percy locally ensures that visual snapshots are correctly generated before integrating into your CircleCI pipeline.
Once your test runs successfully, head over to Percy and create an account. This will allow you to store and compare visual snapshots across different builds.
Next, create a new Percy project.
After creating your new Percy project, a unique Percy project token will be generated for you. This token is required to authenticate your Percy tests within CircleCI, ensuring that Percy can store and compare snapshots across builds.
Go to the CircleCI project you want to integrate Percy on. Click the Project Settings -> Environment Variables -> Add Environment Variables.
Input your Percy token name and the value gotten from your Percy project.
This will link your CircleCI project to Percy.
Finally, update your CircleCI config.yml file with the following configuration:
version: 2.1
orbs:
browser-tools: circleci/browser-tools@1.2.3
jobs:
build:
docker:
- image: cimg/node:20.9-browsers
working_directory: ~/your-project
steps:
- checkout
- browser-tools/install-chrome
- run:
name: Install Dependencies
command: npm install
- run:
name: Start Vite Dev Server
command: npm run dev
background: true
- run:
name: Run Lighthouse CI
command: npx lhci autorun
- run:
name: Install XVFB
command: sudo apt-get install -y xvfb
- run:
name: Run Cypress with Percy
command: npx percy exec -- npx cypress run
Once your changes are made, push them to GitHub:
git add .
git commit -m "your message"
git push origin main
There you have it, Percy is now part of your CircleCI pipeline.
Now, visit your Percy project dashboard to review visual snapshots. Compare the changes made, and approve or reject updates before deployment.
Deployment automation: from preview to production
Manually deploying front-end projects can be a hassle, as the process is often error-prone and time-consuming, making it inefficient for developers who frequently push updates. Fortunately, deployment can now be automated using tools and systems that move code changes between testing and production environments, eliminating the need for manual intervention.
Some key advantages of automated deployment include:
-
Speed and efficiency: Automated deployment significantly reduces the time needed to push updates for production. What used to take hours during manual deployment can now be done in a matter of seconds when deployments are automated, improving productivity for teams managing multiple front-end projects.
-
Fewer errors: Manual deployments often involve multiple steps, increasing the risk of human error. With automated deployment, these errors are drastically reduced, making deployment as error-free as possible.
-
Simplified deployment process: Manual deployment of front-end applications often requires a specific level of skill set that not every developer possesses. However, automated deployment simplifies the process, making it more accessible even for those with minimal deployment experience.
With CircleCI, you can automate deployments to Vercel, making it easy to push changes from preview to production.
Setting up CircleCI to automate deployment to vercel
To integrate Vercel into your CircleCI pipeline, start by creating a Vercel account.
Next, create a new project and import the GitHub repo you want to automate deployment on.
Name your project and click Deploy.
After deployment, head over to your Vercel account settings > tokens to create a Vercel token.
Next, move to your CircleCI Project Settings -> Environment Variables -> Add Environment Variables.
Add your created Vercel token to your CircleCI project environment variable.
Finally, update your CircleCI config.yml as shown below:
version: 2.1
orbs:
node: circleci/node@5
browser-tools: circleci/browser-tools@1.2.3
jobs:
build:
executor: node/default
steps:
- checkout
- node/install-packages:
pkg-manager: npm
- run:
name: Create the ~/artifacts directory if it doesn't exist
command: mkdir -p ~/artifacts
# Copy output to artifacts dir
- run:
name: Copy artifacts
command: cp -R build dist public .output .next .docusaurus ~/artifacts 2>/dev/null || true
- store_artifacts:
path: ~/artifacts
destination: node-build
- browser-tools/install-chrome
- run:
name: Install Dependencies
command: npm install
- run:
name: Start Vite Dev Server
command: npm run dev
background: true
- run:
name: Run Lighthouse CI
command: npx lhci autorun
- run:
name: Install XVFB
command: sudo apt-get install -y xvfb
- run:
name: Run Cypress with Percy
command: npx percy exec -- npx cypress run
preview:
executor: node/default
steps:
- checkout
- run:
name: Install Vercel CLI
command: npm install -g vercel
- run:
name: Deploy project to Vercel (preview)
command: vercel --token $VERCEL_TOKEN --yes
deploy:
executor: node/default
steps:
- checkout
- run:
name: Install Vercel CLI
command: npm install -g vercel
- run:
name: Deploy project to Vercel
command: vercel --token $VERCEL_TOKEN --prod --yes
workflows:
version: 2
preview_deployment:
jobs:
- build
- preview:
requires:
- build
filters:
branches:
ignore: main
- deploy:
requires:
- build
- preview
filters:
branches:
only: main
The code above runs two jobs: preview and deploy. When changes are pushed to a GitHub branch, the preview job is triggered. However, when changes are pushed to main, the deploy job gets executed which means your application gets deployed.
Congratulations! You now have CircleCI automating the deployment of your front-end project.
With your integration steps now complete, here’s the full CircleCI config.yml
file combining CI/CD, Lighthouse CI, Percy, and automated deployment to Vercel. You can also find the complete file on GitHub.
Conclusion
So far, you have learned how to implement DevOps practices in front-end development by setting a CI/CD pipeline in CircleCI. Your pipeline automates performance and accessibility testing with Lighthouse CI, visual regression testing with Percy, and automates deployment to Vercel.
By integrating these workflows, you can improve your code quality, enhance team collaboration, and maintain reliable front-end projects.
I encourage you to implement all that you have learned in both your new and existing projects. Also, explore the official documentation of CircleCI, Lighthouse CI, Percy, and Vercel to deepen your understanding and further optimize your workflow.