Display your CircleCI build status from on Jira
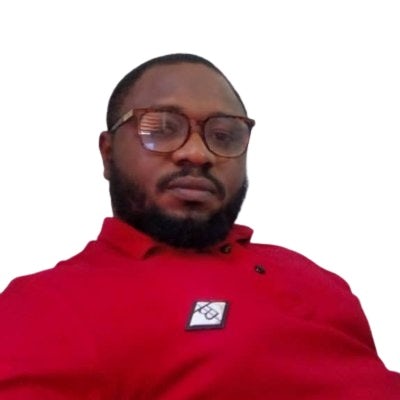
Fullstack Developer and Tech Author
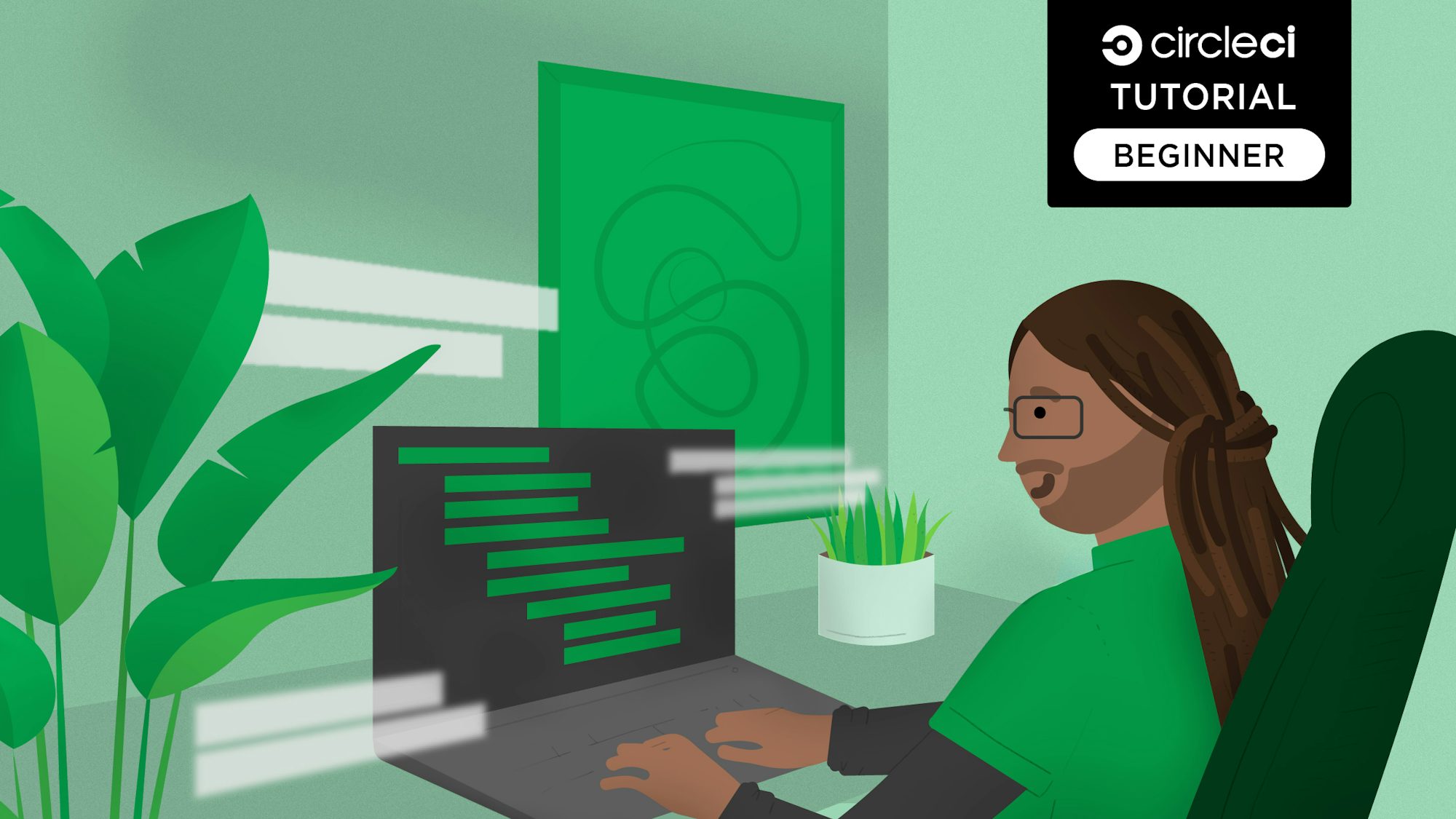
Automating tests and deployments with CI/CD makes development more productive. Instead of managing multiple tools and manual processes, all they have to do is commit the code to a code repository.
Not everyone on a project manages the CI/CD system, but they may need to know when the build process fails or is successful. This is where a proper handshake between CI/CD systems and project management tools such as Jira shines.
Jira is well known and used by many, if not most, software development teams as a project management software for tracking issues, managing Scrum and Agile projects, and more.
In this tutorial, I will show you how to set up integration between your CI/CD workflows (CircleCI for this project) and Jira work items. With this implementation, you have access to the status of all your builds and deployments right within your Jira panel. To make this integration happen, you will use the Jira orb from the CircleCI orb registry.
Prerequisites
For this tutorial, you will need:
- Node.js installed on your computer
- A GitHub account
- A CircleCI account
- A Twilio account
- An Atlassian account
- Admin permissions on JIRA
Getting started
To monitor the status of your workflow, you will build a basic Node.js application that returns a message from the homepage route /
. You will also write a test assert that the expected message was returned.
Create a folder for the project and move into the newly created folder using this command:
mkdir node-jira-application && cd node-jira-application
Next, create a package.json
file with dynamically defined values within the project:
npm init -y
Installing project dependencies
To build the application, you will use Express as the Node.js express web application framework. Run this command to install Express:
npm install express --save
We will use Jest as the testing framework for the project. Install Jest with this command:
npm install --save-dev jest supertest
Once the installation process is completed, create two new file named index.js
and server.js
within the root of the project:
touch index.js server.js
Open the new file and populate it with this content:
Use the following content for index.js
:
var express = require("express");
var app = express();
// Root URL (/)
app.get("/", function (req, res) {
res.send("Sample Jira App");
});
module.exports = app;
And paste the following in server.js
:
const app = require("./index");
//server listening on port 8080
const PORT = process.env.PORT || 8080;
const server = app.listen(PORT, () => {
console.log(`Listening on port ${PORT}`);
});
module.exports = server;
The content you added specifies the message that should be displayed at the homepage of the project.
Next, run the application with:
node server.js
From your browser, go to http://localhost:8080
.
The result is just a basic message to confirm that your application works. Stop the application from running by typing CTRL + C
in your terminal. Press Enter to proceed.
Create a test script
To get started, create a separate folder within the application to house the test scripts. Name it test
.
mkdir test
Add a new file to the test
folder and name it test-home.spec.js
:
touch test/test-home.spec.js
Next, add a simple test to confirm the home page contains the text “Sample Jira App”. Paste this content into the file:
const request = require("supertest");
const app = require("../index");
let server;
beforeAll(() => {
server = app.listen(0);
});
afterAll((done) => {
server.close(done);
});
describe("Home page content", () => {
it('should return "Sample Jira App"', async () => {
const response = await request(server).get("/");
expect(response.text).toBe("Sample Jira App");
});
});
Run the test locally
First, update the scripts
section in the package.json
file with a command that runs both the application and test script easily. Enter this content:
{
"name": "jira-app",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "jest",
"start": "node index.js"
},
...
}
}
Run the application with npm run start
. From a different tab but still within the project, issue this command to run the test:
npm run test
This will be the result:
➜ node-jira-application npm run test
> jira-app@1.0.0 test
> jest
PASS test/test-home.spec.js
Home page content
✓ should return "Sample Jira App" (12 ms)
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Snapshots: 0 total
Time: 0.264 s, estimated 1 s
Ran all test suites.
Adding CircleCI configuration to automate the test
To automate the test, create a .circleci
folder inside the root directory of your application. Add a new file named config.yml
to it. Open the newly created file and paste this code:
version: 2.1
orbs:
node: circleci/node@7.1.0
jobs:
build-and-test:
executor:
name: node/default
steps:
- checkout
- node/install-packages
- run:
name: Run the app
command: npm run start
background: true
- run:
name: Run test
command: npm run test
workflows:
build-and-test:
jobs:
- build-and-test
This configuration file specifies which version of CircleCI to use. It uses the CircleCI Node orb to set up and install Node.js. The config also installs all the dependencies for the project.
The final command is the actual test command, which runs your test.
Before you deploy the project to GitHub, create a .gitignore
file in the root directory of your project. Enter this:
# compiled output
/dist
/node_modules
# Logs
logs
*.log
npm-debug.log*
yarn-debug.log*
yarn-error.log*
lerna-debug.log*
# OS
.DS_Store
# IDEs and editors
/.idea
.project
.classpath
.c9/
*.launch
.settings/
*.sublime-workspace
# IDE - VSCode
.vscode/*
!.vscode/settings.json
!.vscode/tasks.json
!.vscode/launch.json
!.vscode/extensions.json
Now you can push the project to GitHub.
Connecting the application to CircleCI
At this point, you need to connect the application to CircleCI. To do that, log in to your CircleCI account with the linked GitHub account that contains this application. Go to the Projects page on the CircleCI dashboard, search for the project and click Set Up Project.
Using the Fastest option, enter the name of the branch housing your config file.
and click Set Up Project.
This will trigger the pipeline and the build should run successfully
Connecting Jira with CircleCI
Enabling successful interaction between CircleCI and Jira software requires few steps. Here they are in order:
- Create a project and Issue on Jira.
- Install CircleCI for Jira plugins.
- Add Jira token to CircleCI.
- Create a Personal API token on CircleCI.
- Use CircleCI version 2.1 at the top of your
.circleci/config.yml
file. - Add the orbs stanza below your version, invoking the Jira orb.
- Use
jira/notify
command in your existing jobs to send status to the Jira development panel.
Create a Jira project and issue
Log in to your Jira instance and create a project. On the project page, click the Create button.
Give it a name that best describes the issue. For this tutorial, use Build Status Demo App Issue
.
When the issue is created, you can review it in detail by clicking on the issue in the list.
Be mindful of the key for this issue; it will be used to uniquely identify the issue in CircleCI.
Install CircleCI Jira plugins
Navigate to your Jira dashboard and explore the Marketplace. Search for the CircleCI for Jira app.
Note: You must be a Jira admin to install the plugins.
Click, Get now then follow the instructions to install the plugins and set them up.
Once successfully installed, click Configure to be redirected to the app configuration page.
From the configuration page, click Allow access to grant the app access to your Jira instance. Next, you will need to provide your CircleCI Organization ID, which you can find on your CircleCI dashboard by navigating to Organization Settings -> Overview in the CircleCI application
Once you have provided your CircleCI Organisation ID, click Submit to save your changes.
Copy your Webhook URL from the configuration page. This URL is used to send build status updates to Jira.
Configure the Jira Orb on CircleCI
The circleci/jira
orb is used to send build status updates to Jira. To use the orb, you need to add a Webhook URL from previous section to your CircleCI project. Do this by clicking Project settings from the sidebar, then environment variables and add a new variable named JIRA_WEBHOOK_URL
with the Webhook URL as its value.
Enabling CircleCI for Jira software
Before you can start showing the status of your builds from CircleCI on Jira, you need to make some modifications to the CircleCI configuration file.
Back in your project, open .circleci/config.yml
and update its content by adding the Jira orb:
version: 2.1
orbs:
node: circleci/node@7.1.0
jira: circleci/jira@2.2.0
jobs:
build-and-test:
executor:
name: node/default
steps:
- checkout
- node/install-packages
- run:
name: Run the app
command: npm run start
background: true
- run:
name: Run test
command: npm run test
workflows:
build-and-test:
jobs:
- build-and-test:
post-steps:
- jira/notify:
pipeline_id: << pipeline.id >>
pipeline_number: << pipeline.number >>
We included a Jira orb and the workflow as well. The jira/notify
command will be used to send the status of builds to the Jira instance.
Update Git and push the project back to GitHub. This time, create a features branch using the key for the task or issues id on Jira. In this case it is PBSD-1
.
git checkout -b features/PBSD-1
Do not forget to replace PBSD-1
with your key if it is different.
From the sidebar menu you can click into the build or deployment information to view the CircleCI job details.
Conclusion
Among other benefits, connecting CircleCI to Jira software keeps everyone in your development team up-to-date with the status of every build without needing to go to the pipeline dashboard on CircleCI.
In this tutorial, you:
- Built a simple Node.js application
- Wrote a test script for it
- Followed the steps to connect a CircleCI pipeline with a Jira item
- Displayed the status of the CircleCI builds on Jira
I hope you found this tutorial helpful. If you did, share it with your team!
The complete code for this tutorial can be found on GitHub.