Automating CSS code quality in front-end projects with Stylelint and CircleCI
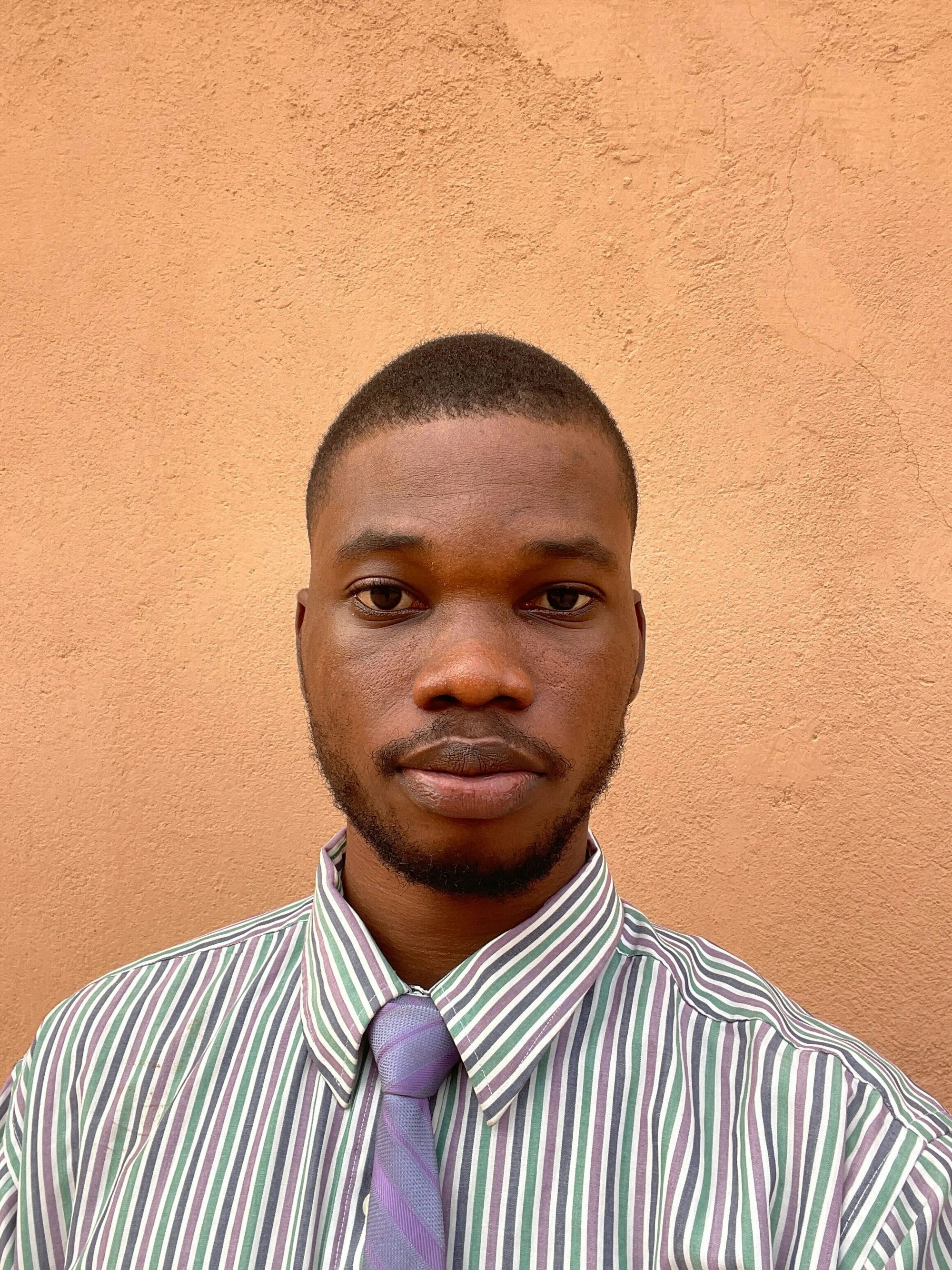
Front-end Developer
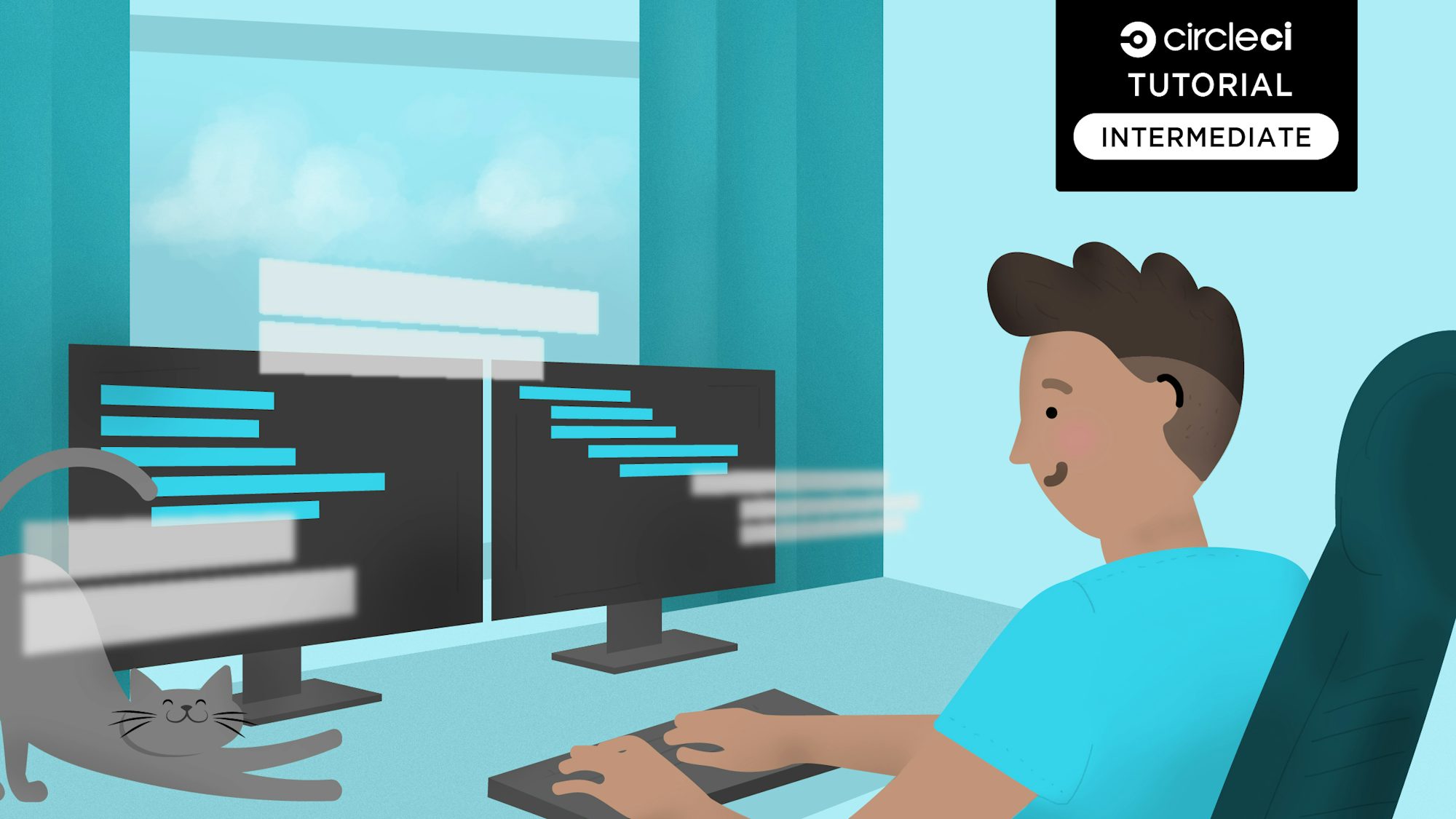
Cascading Style Sheets (CSS) is the language used by developers to apply styles to documents written in a markup language. In front-end development, enforcing consistent CSS code quality is crucial: poorly written CSS can lead to issues ranging from poor maintainability, unexpected bugs, and inconsistent designs. One effective way to ensure CSS code quality is using a linter such as Stylelint.
Stylelint is a static analysis tool that analyzes CSS and preprocessors like the Syntactically Awesome Stylesheet (SCSS) for errors in styles and conventions. It does this by detecting invalid CSS syntax, enforcing indentation and spacing rules, ensuring proper and consistent naming conventions, and flagging missing semicolons, among other issues. In essence, Stylelint is the perfect solution for improving CSS code quality.
In this article, you will learn how to set up Stylelint in a front-end project, integrating it into a CircleCI pipeline to automate CSS code quality enforcement.
Prerequisites
To follow along with this article, you will need:
- A knowledge of React.
- A GitHub account.
- Install Node.js 18.17 or above
- A CircleCI account.
Pushing existing front-end project to GitHub
To automate CSS code quality using Stylelint and CircleCI, your project needs to be version-controlled with Git and pushed to a GitHub repository.
This tutorial uses a simple blog card component built with React as the front-end project. To get started, clone the repository locally using the following command:
git clone https://github.com/CIRCLECI-GWP/stylelint-react-blog-card-circleci
cd stylelint-react-blog-card-circleci
git checkout starter
Note: You could also follow along this tutorial using a different codebase and automate CSS code quality in it using Stylelint and CircleCI.
To initialize Git in your project and push it to a GitHub repository, follow the steps in the linked blog post, which is an excellent guide if you have little knowledge of GitHub.
Setting up Stylelint
With your project now pushed to your GitHub repository, it is time to ensure CSS code quality with Stylelint.
To set up Stylelint, start by installing it using the following command in your terminal:
npm install --save-dev stylelint stylelint-config-standard
Next, create a Stylelint configuration file in your project directory. You can do this manually by creating a stylelint.config.js
file or by running the below command in your terminal:
touch stylelint.config.js
Within your stylelint.config.js
file, add the following code:
export default {
extends: ["stylelint-config-standard"],
rules: {
"unit-allowed-list": ["px", "rem", "%", "ms"],
"selector-max-id": 0,
"color-named": "never",
}
}
The above code sets up a basic Stylelint configuration with a few rules.
Note: The rules used in this tutorial are basic rules that improve code quality in CSS
-
The
unit-allowed-list
rule enforces consistent use of specific units across your CSS code. -
The
selector-max-id
rule prevents the use of ID selectors in your CSS code, which is a good practice for code scalability and avoids high specificity issues. -
The
color-named
rule enforces the use of color codes throughout your CSS as opposed to named colors like red or blue.
Note: The rules used in this tutorial are basic rules that improve code quality in CSS. You can further customize other rules in your Stylelint configuration file to match your project needs.
Run Stylelint to check your CSS files:
npx stylelint “path to your css file”
Since this tutorial runs Stylelint on the CSS file in the blog card component, the command will be:
npx stylelint "src/components/Blogcard.css"
Running this command will display any CSS rule violations in your terminal. If no violations are found, the command will not produce any output.
You can also run Stylelint on every CSS file in your porject using the following command:
npx stylelint "**/*.css"
Note: The process is similar to running Stylelint on a single CSS file, but this command will check all .css files in your project, including those in subdirectories.
Finally, commit and push changes to GitHub. You can do this by running the below command on your terminal:
git add .
git commit -m "commit message"
git push origin main
Now that Stylelint is set up and successfully running locally, you can take a step further by integrating it into your CI/CD pipeline with CircleCI.
Integrating Stylelint into CircleCI
To further optimize Stylelint in your project, you can integrate it into a CI/CD pipeline. A great CI/CD tool for this purpose is CircleCI. Integrating Stylelint in CircleCI will ensure that linting rules are adhered to automatically whenever the CircleCI pipeline runs.
Before integrating Stylelint with CircleCI, navigate to your package.json file and add the following script:
"scripts": {
"lint:css": "stylelint 'src/components/Blogcard.css'",
}
Note: The "script": {"lint:css":}
contains the directory to the blog card component CSS file. Ensure to replace it with the directory of your project’s CSS file.
If you want to lint multiple CSS files across your project, use the following script:
"scripts": {
"lint:css": "stylelint 'src/**/*.css'",
}
To integrate Stylelint with CircleCI, navigate to your project directory and create a .circleci
folder at the root. Inside this folder, add a new config.yml
file with the following content:
version: 2.1
jobs:
lint-css:
docker:
- image: cimg/node:18.17
steps:
- checkout
- run:
name: Install dependencies
command: npm install
- run:
name: Run StyleLint
command: npm run lint:css
workflows:
version: 2
lint:
jobs:
- lint-css
In the above configuration, necessary dependencies are installed using the npm install
command. Once the dependencies are installed, Stylelint gets executed using the npm run lint:css
command, analyzing the project’s CSS files and enforcing the defined Stylelint rules in the stylelint.config.js
file.
The entire setup runs inside a Docker container using the cimg/node:18.17
image, ensuring a consistent Node.js environment.
Within your workflow is the lint-css
job, which automates the linting process and triggers the job whenever changes are pushed to the repository.
Commit the changes and push them to GitHub.
Setting up the project on CircleCI
With your .circleci
folder and config.yml
file in place, it is time to set up your project on CircleCI.
To do this, log in to your CircleCI account and navigate to the project’s dashboard. Search for the name of your project repository and click Set Up Project.
Next, enter the Git branch name that contains your .circleci
folder (in this tutorial, that is the main branch), and click Set Up Project to trigger CircleCI to build a pipeline.
Once the setup is complete, the first build will automatically be triggered.
The build will take some time to process, and with everything properly configured, the build should finish successfully.
You can click on the lint
workflow to view the pipeline details.
CircleCI will subsequently trigger builds automatically when new commits are made. Now that CircleCI is set up within your project to run Stylelint, any CSS error in your project will be caught automatically, ensuring consistent styling across your codebase.
Testing the pipeline
With CircleCI now configured to run Stylelint on each commit, the question is what happens if your CSS code violates one or more of the rules specified in your stylelint.config.js
file?
To demonstrate this, introduce some intentional errors in your CSS code that go against the Stylelint rules you previously defined:
Note: The following code is copied from Blogcard.css, which we have been using for this tutorial. Endeavor to make similar changes to the CSS file of your project.
* {
box-sizing: border-box;
font-family: -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, Oxygen,
Ubuntu, Cantarell, "Open Sans", "Helvetica Neue", sans-serif;
}
.card {
width: 400px;
height: 550px;
margin: 50px auto;
background: #141414;
border: solid black 1px;
}
.image img {
background-size: 400px 400px;
background-repeat: no-repeat;
width: 100%;
height: 350px;
margin: auto;
}
.description p {
width: 100px;
height: 35px;
border-radius: 20px;
text-align: center;
padding: 4px 2px;
margin-left: 15px;
background: #9e2222;
font-weight: lighter;
color: #d3d3d3;
}
.content p {
color: #d3d3d3;
margin: 0px 15px;
text-align: justify;
font-weight: lighter;
}
In the modified code above, two rule violations have been introduced:
-
The
.card {border: solid black 1px}
breaks thecolor-named
rule by using a named color (black) as opposed to a color code. -
The
.content p {margin: 0px 15px}
violates theunit-allowed-list
rule by having the top and bottom margin set to zero carrying an unnecessary unit (px).
When these changes are committed and pushed to GitHub, CircleCI triggers the pipeline, and the build fails due to these CSS rule violations.
To fix the errors, update the CSS code to comply with your specified Stylelint rules:
.card {
border: solid #0000 1px; /* Using a hex color instead of a named color */
}
.content p {
margin: 0 15px; /* Removing the unnecessary unit from zero */
}
Once these corrections are made and the changes pushed to GitHub, CircleCI will rerun the pipeline, and the build should now pass successfully.
Conclusion
Improving the quality of your CSS code is not just about aesthetics, but also about code maintainability, scalability, and efficiency. You can enhance your CSS code quality by implementing Stylelint as outlined in this tutorial. In projects with multiple CSS files, relying solely on manually running Stylelint can be tedious.
By integrating Stylelint into a CI/CD pipeline with CircleCI, you can automate the enforcement of consistent styling rules, reduce code errors, and improve team collaboration. This ensures that every commit adheres strictly to the defined CSS standards without manual input.
So, if you haven’t already, consider integrating Stylelint into your project and automating the process to maintain code consistency and reliability across your team.